Vtimes Chrome Plugin
Introduction
Vtimes, similar to MetaMask, is a bridge for allowing Vision DApps to run in the browser without having to deploy a Vision Full Node.
Download
If a user has Vtimes already installed in the Chrome extension , then Vtimes injects a version of VisionWeb into every browser page.
Inject Application
This allows for the web DApp to interact with the Vision Network. Let's learn it with a simple example:
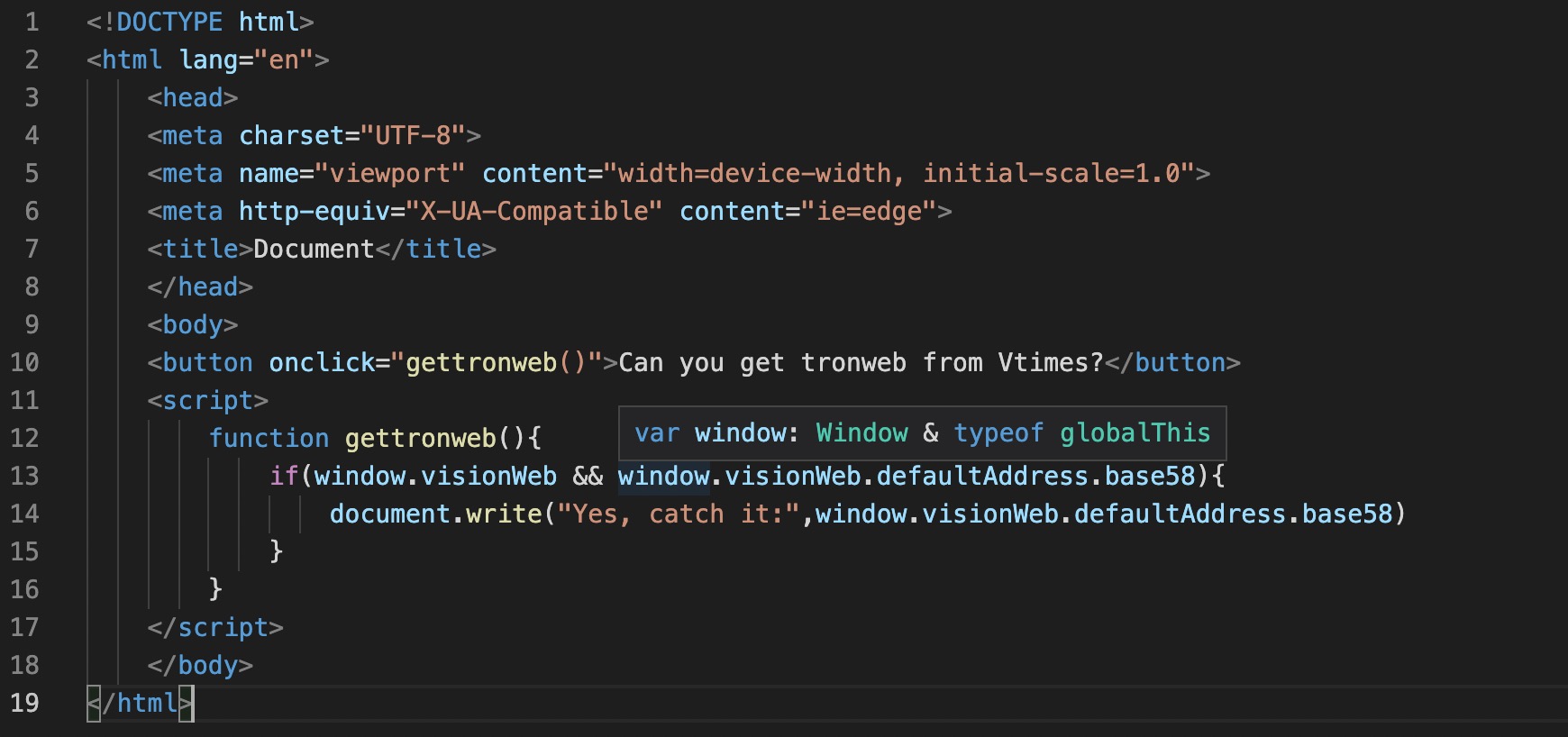
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
</head>
<body>
<button onclick="getVisionweb()">Can you get visionweb from Vtimes?</button>
<script>
function getVisionweb(){
if(window.visionWeb && window.visionWeb.defaultAddress.base58){
document.write("Yes, catch it:",window.visionWeb.defaultAddress.base58)
}
}
</script>
</body>
</html>
Of course,visionweb has many functions waiting for you to use.
Signature
In the process of completing the transaction, visionweb needs to let Vtimes perform the signature. Here Vtimes rewrites the signature. The signature process is completed in Vtimes, and then the signed transaction is returned to visionweb for broadcasting. Let's learn it with a simple example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
</head>
<body>
<script>
var obj = setInterval(async ()=>{
if (window.visionWeb && window.visionWeb.defaultAddress.base58) {
clearInterval(obj)
let visionWeb = window.visionWeb
let vx = await visionWeb.transactionBuilder.sendVs('VN9RRaXkCFtTXRso2GdTZxSxxwufzxLQPP', 10, 'VTSFjEG3Lu9WkHdp4JrWYhbGP6K1REqnGQ')
let signedVx = await visionWeb.vs.sign(vx)
let broastVx = await visionWeb.vs.sendRawTransaction(signedVx)
console.log(broastVx)
}
}, 10)
</script>
</body>
</html>
When the code is executed to visionweb.vs.sign(vx) , Vtimes will pop up a window to confirm the signature.
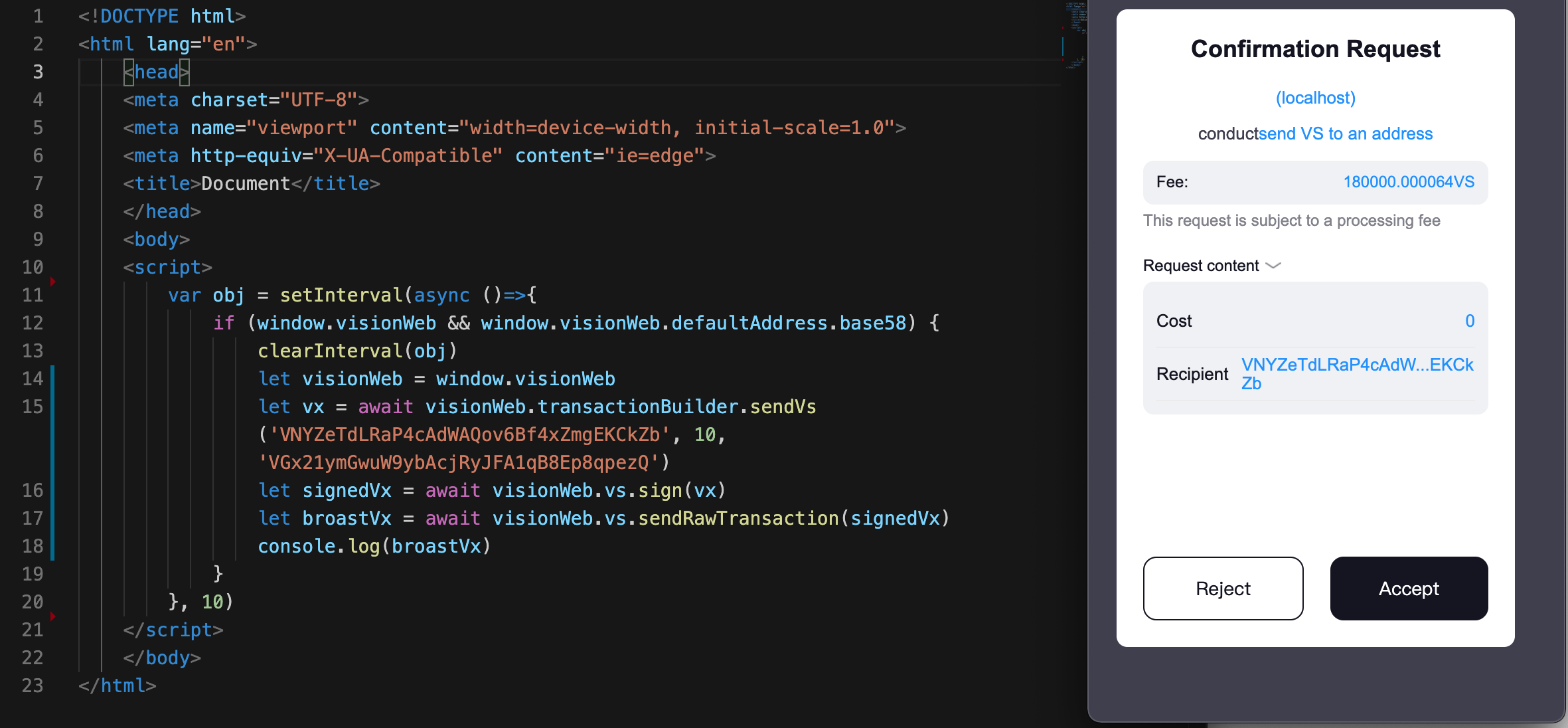
Now, I believe you have been able to complete VisionWeb function calls in conjunction with Vtimes.
Vtimes events
Vtimes currently supports sidechains and mainchains. Developers can detect the event message sent by Vtimes in DAPP, what we can learn from this event message contain the sidechain or mainchain currently selected by Vtimes, and which account is currently selected. Let's learn it with a simple example.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
</head>
<body>
<script>
window.addEventListener('message', function (e) {
if (e.data.message && e.data.message.action == "tabReply") {
console.log("tabReply event", e.data.message)
if (e.data.message.data.data.node.chain == '_'){
console.log("Vtimes currently selects the main chain")
}else{
console.log("Vtimes currently selects the side chain")
}
}
if (e.data.message && e.data.message.action == "setAccount") {
console.log("setAccount event", e.data.message)
console.log("current address:", e.data.message.data.address)
}
if (e.data.message && e.data.message.action == "setNode") {
console.log("setNode event", e.data.message)
if (e.data.message.data.node.chain == '_'){
console.log("Vtimes currently selects the main chain")
}else{
console.log("Vtimes currently selects the side chain")
}
}
})
var obj = setInterval(async ()=>{
if (window.visionWeb && window.visionWeb.defaultAddress.base58) {
clearInterval(obj)
let visionweb = window.visionWeb
}
}, 10)
</script>
</body>
</html>
The above code involves three events: tabReply, setAccount, and setNode. The following are the triggering scenarios of these events:
The completing Vtimes initialization(after page load) | tabReply |
Main chain and side chain switching in Vtimes: | setAccount、setNode |
Setting nodes in Vtimes | setAccount、setNode |
-
Before the DAPP page is loaded, you can judge the data.message.data.data.node.chain field of the tabReply event to determine whether Vtimes chose the side chain or the main chain when the page was loaded. If it is '_', it means the main chain. , Otherwise it is the side chain, and the number of the side chain represented by chain, the number of each side chain is unique.
-
After the DAPP page is loaded, you can judge the data.message.data.data.node.chain field of the setNode event to determine whether the user manually selected the side chain or the main chain in Vtimes. If it is '_', it means the main chain , Otherwise it is the side chain, and the number of the side chain represented by chain, the number of each side chain is unique.
Updated almost 3 years ago